Posts Tagged ‘debugging’
July 7th, 2017
I seldom use F5 (start the application in debugging mode in Visual studio) but instead run it normally and connect the debugger only when I need to.
Connecting the debugger takes a measurable amount of time so with a faster turnaround I can keep focused better.
I also leave IIS running all the time. It does shadow copying so there is no need to restart anything.
I have recently switched from IIS to IIS express while developing and hence need to have it running all the time. That I do through the command prompt with
1
| "c:\Program Files\IIS Express\iisexpress.exe" /path:C:\PathToMyWeb /port:32767 |
where path is the path to the site (where the web.config resides) and the port is what you see in the web project properties or in the web browers’s address bar the first time you hit F5.
It has the nice side effect that I can see all the http requests I do in the command window, a light weight current log of sorts.
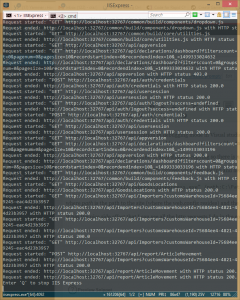
September 6th, 2014
I finally managed to write through Debug.WriteLine to Sysinternals DebugView through reading this article. Kudos to the author.
If the original article disappears; here is the recipe in short:
Start Sysinternals Debugview as administrator. Make sure Capture global win32 is checked.
Make sure Visual studio is not attached as debugger because when you run the VS debugger it hogs all information. (In VS you can find the output in the Output window with Show output from Debug.)
Besides Debug.WriteLine also play around with Trace.WriteLine and Console.WriteLine.
March 7th, 2013
When debugging and watching an object one only sees the name of the type in the debugger.
1
| MyProjectNamespace.Meeting |
The quickest solution is to click the little plus sign or use the keyboard right arrow to show the properties. This is ok to do once or twice but doing this for every comparison is a waste of time at best.
Better then is to use SystemDiagnostics.DebuggerDisplay like so:
1 2 3
| [DebuggerDisplay("ID:{ID}, UID:{UID}, Name:{Name}, Type:{this.GetType()}")]
public class Meeting : BaseClass {
... |
to get
1
| ID:1, UID:234abc, Name:XYZ, Type:MyProjectNamespace.Meeting |
The Tip was to use {this.GetType()} in the argument of DebuggerDisplay.
Update
To show count of lists use something in the lines of:
1
| [DebuggerDisplay("ID:{ID},Customers:{Customers==null?(int?)null:Customers.Count}")] |
Update update
Since the DebuggerDisplay parameter is written as is into the assembly it might not work between languages. A solution for this is to call a method instead as most lanuguages uses the MyMethod() syntax.
It is also creates less overhead to call a method instead of having the expression in a string according to the MSDN link below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| [DebuggerDisplay("{DebuggerDisplay(),nq}")]
public class ExchangeRateDto
{
public int ID { get; set; }
public string Name { get; set; }
#if DEBUG
private string DebuggerDisplay()
{
return
$"ID:{this.ID}, Name:{this.Name}, Type:{this.GetType()}";
}
}
#endif |
Update update update
Use nameof. Also skip the this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| [DebuggerDisplay("{DebuggerDisplay(),nq}")]
public class ExchangeRateDto
{
public int ID { get; set; }
public string Name { get; set; }
#if DEBUG
private string DebuggerDisplay()
{
return
$"{nameof(ID)}:{ID}, {nameof(Name)}:{Name}, Type:{GetType()}";
}
}
#endif |
Update update update update
I have noticed that in Visual Studio 2022 (possibly earlier) one can ctrl-. on the class or record name and Visual studio writes the boilerplate code. The DebuggerDisplay is called GetDebuggerDisplay and only returns this.ToString() unfortunately. So it has to be adapted.
—
Praise where praise is due: https://blogs.msdn.microsoft.com/jaredpar/2011/03/18/debuggerdisplay-attribute-best-practices/.
There is more documentation at Stack overflow documentation.
Search Term: DebuggerDisplayAttribute
September 2nd, 2010
If you are working with CLR in SQLServer you might run into
A .NET Framework error occurred during execution of user-defined routine or aggregate "MyCLRMethod": System.InvalidOperationException: The context connection is already in use.
I cannot say this is the solution every time but it worked for me. Look down to the top of the stack (furthest down in the text) and you get some more clues.
Msg 6522, Level 16, State 1, Line 1
A .NET Framework error occurred during execution of user-defined routine or aggregate "MyCLRMethod":
System.InvalidOperationException: The context connection is already in use.
System.InvalidOperationException:
at System.Data.SqlClient.SqlInternalConnectionSmi.Activate()
at System.Data.SqlClient.SqlConnectionFactory.GetContextConnection(SqlConnectionString options, Object providerInfo, DbConnection owningConnection)
at System.Data.SqlClient.SqlConnectionFactory.CreateConnection(DbConnectionOptions options, Object poolGroupProviderInfo, DbConnectionPool pool, DbConnection owningConnection)
at System.Data.ProviderBase.DbConnectionFactory.CreateNonPooledConnection(DbConnection owningConnection, DbConnectionPoolGroup poolGroup)
at System.Data.ProviderBase.DbConnectionFactory.GetConnection(DbConnection owningConnection)
at System.Data.ProviderBase.DbConnectionClosed.OpenConnection(DbConnection outerConnection, DbConnectionFactory connectionFactory)
at System.Data.SqlClient.SqlConnection.Open()
at Elvis.DatabaseCLR.UserDefinedFunctions.GetNextSampleID(DateTime readDate, Int32 meterID, Boolean useNextMeter)
at Elvis.DatabaseCLR.UserDefinedFunctions.ConsumptionForNewMeter(Int32 sampleID, DateTime readDate, Int32 meterID, Decimal meterCoefficient, Int32 previousMeterConsumption, DateTime presentationDateOfSample)
at Elvis.DatabaseCLR.UserDefinedFunctions.MyCLRMethod(Int32 sampleID, DateTime presentationDate, DateTime readDate, Int32 meterID, Decimal meterCoefficient, Boolean newMeter)
.
The MyCLRMethod calls two more methods until Open is called. Playing around I found that I couldn’t open a connection if one already was open. It sounds right since one of the deals with CLR in SQLServer is that we already have a connection. The resolution is to tidy up the code so we only open the connection once, or open/closes it over and over again.
Tricks I used:
– When you get the error in your applicaiton copy the SQL string to your query editor and run it from there.
– Press F5 in Visual studio and have Visual studio deploy the CLR for you. But use the query tool to run the query. The error message is the same.
– Debug-through-printf, or “throw exception” in this case. Drop in a throw-exception to find out exactly which Open call is failing. The F5-and-wait and then run query takes a while. If you have a logging possibility it is handy now.