Posts Tagged ‘architecture’
September 27th, 2016
I have found out that Automapper needs to be set up once and only once.
This means that one place has to know of all the classes that have a mapping. In a multi tier application this is not possible without treading on someone’s toes.
I have seen a solution which loops through all loaded assemblies and maps once and for all. But this solution means we have one such point – like when a web app is loading. So if you have automatic tests, that setup has to be called from there. And any kind of automatic loading of assemblies cannot use Automapper. Well… if one reinitialises all mappings one can.
I might see another solution where one has one assembly that knows of all assemblies (with mapping) and makes sure Automapper is initialised. My experimentation with this lead to circular reference problem though.
When I am at it – the documentation is short and straight to the point (good) but misses the point that the code in the documentation should be usable (not good).
The API was not explorable, which means that there is no natural way to start mapping and find out how the API works as one goes. Instead I had to google and find a bit here and a bit there.
With that said – it still solved a problem for me. And that is considered good.
June 5th, 2014
Abstract
The only way to edit architecture and modelling with Visual studio is to buy the very expensive Ultimate edition. This means that it will be not everyone can be involved in the process of evolving the solution.
TL;DR
Development requires Architecting an Architecture in some way or another. This architecture is not written in stone but changes as time or business changes; we call it requirements change.
In the old days, like 20 years ago, I learned that there is an architecture phase and then a development phase. This is not considered best practice any more. Also not best practice is to have silos with Architectures and Testers and Coders and Database managers.
This is where Microsoft and Visual studio fails. Not technically but license wise.
Look at this part of a comparison chart for different Visual studio versions. It clearly explains that Architects must have the Ultimate edition and that no one but Architects are allowed to change any Architect document. (the Ultimate edition is way more expensive than the others)
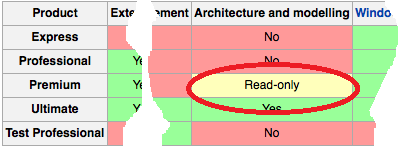
Visual studio comparison fail where it shows that only Ultimate can edit architecture stuff. Original from Wikipedia.
May 24th, 2013
TL;DR
A domain model does not have to look like the database schema.
They can resemble each other but they don’t have to.
A domain model is for handling domain problems while a database is for storing and retrieving data. Let each tool do what it is best at; a hammer for nails and a screw driver for screws.
May 13th, 2008
I like unit tests, I really do. But I have also come to realize that many tests don’t have to be done.
One can for instance code according to Fail At Once to make the compiler do the tests.
Or one can autogenerate code and hence only have to unit test a fraction of the resulting code.
Or one can do integration tests and take for granted that if the integration works the unit tests work too.
I have tried all three and believe in laziness – to get more done while working less. So I say that a thought out mix of all three above supposedly is the right way.
Patrik Löwendahl thinks approximately the same.
September 25th, 2007
I found a blog entry at http://www.matshelander.com/wordpress/?p=74 which although lengthy and wordy hits the spot. There is no good notation system for software architecting.
My words: Comparing software architecting to building houses is ridiculous. Just because they share the same name (architecting) doesn’t mean they have a lot in common. Compare a sea horse and a horse and you get the same useless result.
The romans would never be good at mathematics since they lacked a good notation.
What software architecting needs is a good notation. UML is one way but it is not very good for evolving solutions and finding bugs. Code is the best notation I know of but it is too close to the very program and not good at describing architecture.
Besides, after several thousand years of building houses we still miss the estimated cost.
September 21st, 2007
We are used to having PKs in databases as integers.
The reason for this is that computers are good at numbers and an integer is a very versatile and fast number representation.
But there are drawbacks in the business layer. A customerID can be compared to a orderID like so:
if( customerID == orderID ){…
This is not a good thing.
CustomerIDs should only be comparable to other CustomerIDs.
They should also not be addable, subtractable, multiplyable and being greater than anything else. A CustomerID is equal or not equal to another CustomerID. All other comparisions are not of this world.
I have yet to figure out how to implement this.
A comment of what to use as a primary key is found here.
June 17th, 2007
The discussion whether to design software bottom up och top down died with intellisense/typeahead/whateveryoucallit; it was so much easier building bottom up. Then refactoring tools entered and suddenly made it easy to program top down again.
Personally I believe the two methods should be mixed. Not like “these methods or modules we design top down and these bottom up” but more like “these methods we design no matter the way”.
Like this:
Here is a method I’d like to have. Here is the call. There is the method signature automagically created. Then some implementing code. And further calls to yet non-existing methods. Which are automagically created. Until we get all the way to the bottom. Where we adjust a couple of parameters. And refactor this up again. And adjust. And refactor. Until we are back where we started with code that is readable both if you track it from the top or the bottom.
Or the other way around if you prefer.
As long as the code gets readable. And updatable.
June 17th, 2007
Anything simple.
Anything simple but not something the user knows about.
A 32 bit integer, a GUID, a string. But not the CustomerNumber or PostalCode or EmailAddress. No matter what the user/customer/client says – those things are not unique. I have been harrassing customers whether the CustomerNumber is unique or not. It has been unique for two weeks until someone remembered about foreign offices with their own numbering system or that they sometimes are changed.
Alas – do not use anything you find in the business logic as primary key.
A more technical comment on why we use int is found here.
June 1st, 2007
I talk about the three different roles like this:
– Programming: coding, implementing algorithms.
– Designing: deciding algorithms, choosing protocols, constructing class relations.
– Architecting: making the code and machines work with the business rules.
When a programmer selects to write one piece of code instead of another he makes a (hopefully) aware choice of which code will be the best today and tomorrow. (These two aren’t necessarily the same.) By this code he influences the ways algorithms will be implemented.
When a designer selects a protocol for another he also sets some technical limits. Different protocols are more or less easy to update, debug and trace. This influences the architecture.
When the architect decides for something he has to consider why it should be done, how and by who. (whom?) Every architectural decision must be implemented (or better – does not have to be implemented) somehow and this leads to a design decision in turn.
Now, when the designer decides to use a solution it has to be implemented. It can only be implemented by the programmers. Considerations must be taken for why, done and who.
This boils down to a programmer has to know how to design. A designer must know how to architect. An architect must know how to design. And a designer must know how to program.
I would also say that a good programmer knows how to architect and an architect how to program.
Code written might have architectural implications.
Even comments have design implications.
June 1st, 2007
Strong typing gets rid of lots of runtime errors. Why do script languages always use weak typing? I really don’t see anything good coming out of it.
A friend of mine, mainly a Java and Python programmer, defended Python’s/scriping’s position by telling me how fast it is. He compared the turnaround for a Java web project and a Python ditto. So I see his point, it was quite cumbersome to update the Java solution (I don’t remember the environment), but I have yet to find such a complex situation in any solution I have built for dotnet or VB6.
I doubt all Java solutions are as complex as his example.
Which sort of gets me back to the first paragraph. There is no reason for a scripting language to be weakly typed and hence more error prone.