Posts Tagged ‘asp.net’
July 7th, 2017
I seldom use F5 (start the application in debugging mode in Visual studio) but instead run it normally and connect the debugger only when I need to.
Connecting the debugger takes a measurable amount of time so with a faster turnaround I can keep focused better.
I also leave IIS running all the time. It does shadow copying so there is no need to restart anything.
I have recently switched from IIS to IIS express while developing and hence need to have it running all the time. That I do through the command prompt with
1
| "c:\Program Files\IIS Express\iisexpress.exe" /path:C:\PathToMyWeb /port:32767 |
where path is the path to the site (where the web.config resides) and the port is what you see in the web project properties or in the web browers’s address bar the first time you hit F5.
It has the nice side effect that I can see all the http requests I do in the command window, a light weight current log of sorts.
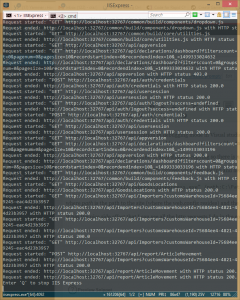
January 14th, 2017
Prerequisite
OSX
Docker
Dotnet core 1.1
A folder named (e.g.) /Users/yourname/Docker/Dandelion
and a folder named (e.g.) /Users/yourname/Docker/Dandelion/MyWeb
Create the web
In MyWeb run
1 2 3 4
| dotnet new -t web
dotnet restore
dotnet build
dotnet publish |
Now we have a web site compiled and ready to run with [dotnet run].
You can run the app and then [curl localhost] to find out if it is runnable; but if you got no error message earlier all should be ok.
Create an image
Go to the Dandelion folder.
Create a file Dockerfile and paste into it:
1 2 3
| FROM microsoft/dotnet
EXPOSE 80
ENV "ASPNETCORE_URLS=http://+:80" |
Then, to create the image run
1
| docker build -t yourname/dandelion . |
Note that the name must be all small caps.
Don’t miss the trailing period.
If you want to check that the image is created just execute [docker images] which should show the new image at the top of the list.
Start the container
Go to the Dandelion folder. (you are probably already standing there)
Execute
1 2 3 4 5
| docker run -p 80:80 \
-ti --rm \
-v /Users/yourname/Documents/Docker/Dandelion/MyWeb/bin/Debug/netcoreapp1.1/publish:/MyWeb \
yourname/dandelion \
/bin/bash -c 'cd /MyWeb; dotnet MyWeb.dll' |
and your web should start.
Verify result
Either open another console and execute
or go to localhost in your web browser.
January 7th, 2017
Prerequisite:
Docker and Dotnet Core 1.1 should be installed on the host (=OSX).
You have a folder somewhere, for instance /User/yourname/Documents/Docker/mywebapp.
Create the image
In your folder, create the dockerfile
1 2 3 4 5 6 7 8
| FROM microsoft/dotnet
# VOLUME /Documents/Docker/mywebapp
EXPOSE 80
ENV "ASPNETCORE_URLS=http://+:80"
RUN mkdir app
WORKDIR /app
RUN dotnet new -t web
RUN dotnet restore |
Then execute
1
| docker build -t mynick/myimage . |
in a terminal in said folder.
If you now execute
you can se the new image as mynick/myimage.
Create the container and start the web server
In the terminal execute
1 2 3 4
| docker run \
-p 80:80 \
-ti --rm mynick/myimage \
/bin/bash -c 'cd /app; dotnet run;' |
Behold result
In a terminal execute
or in a web browser go to