July 19th, 2017
I have one bat file that does all this for me.
It also has a pause inserted so I can visually check that everything looks ok.
The pause between uninstall and install is important as that is where I exchange the DLL when updating.
Yes, the code is ugly. But it has served a project for 10+ years with the only update with new dotnet versions.
I have a similar solution here. It uses several files but can also name the service.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| rem *** Stop the service.
net stop "My Service Name"
pause
rem *** Uninstall the service.
C:\Windows\Microsoft.NET\Framework\v4.0.30319\installutil.exe /uninstall MyService.exe
pause
rem *** Install the service.
C:\Windows\Microsoft.NET\Framework\v4.0.30319\installutil.exe MyService.exe
pause
rem *** Start the service.
net start "My Service Name"
pause |
July 7th, 2017
I seldom use F5 (start the application in debugging mode in Visual studio) but instead run it normally and connect the debugger only when I need to.
Connecting the debugger takes a measurable amount of time so with a faster turnaround I can keep focused better.
I also leave IIS running all the time. It does shadow copying so there is no need to restart anything.
I have recently switched from IIS to IIS express while developing and hence need to have it running all the time. That I do through the command prompt with
1
| "c:\Program Files\IIS Express\iisexpress.exe" /path:C:\PathToMyWeb /port:32767 |
where path is the path to the site (where the web.config resides) and the port is what you see in the web project properties or in the web browers’s address bar the first time you hit F5.
It has the nice side effect that I can see all the http requests I do in the command window, a light weight current log of sorts.
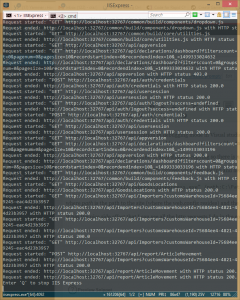
June 17th, 2017
The question is not “Can you do x before month’s end?”.
The question is “Can you remove something that is less important than x?”
In an earlier project I had all my tasks on a wall so when someone wanted a task done they had to physically remove a note and instantly knew that another task would not be done (until something else was done or removed).
To put even more stress on the person, let’s call him Andersson, wanting to allot some of my time the notes/tasks had different owners. This meant that Andersson got a physical feeling that his/her work was more important than someone else’s.
“Do you think I can remove Svenssons note?”
“I don’t know. Ask him.”
This meant I didn’t have to switch task and search out Svensson and present someone else’s task and then come back to Andersson with a result.
Andersson understood his/her action had impact on two other people. Andersson’s problem was still in his/her hands.
My time spared. Svensson’s time spared.
Andersson didn’t have to wait for a decision from someone else.
All win.
June 2nd, 2017
I am presently using TFS’s code reviewing tool and IMHO it lacks visualisation of which comments are important and which are not.
So I came up with the idea of prefixing my comments.
PRAISE: Good refactoring, I like how you remove the negation.
FIX: A null check is missing.
SUGGESTION: Split line.
CHECK: Is it the right enum used here?
EXPLAIN: Is this comparison really correct? or seems to overflow to me.
If there are many FIX but only one or a few are critical I prefix the critical once with RED, like RED FIX.
This gives the opportunity to “while you already are updating the module, do these things too”.
When code reviewing do not forget to give appraisal too
Update:
This is what I wrote at Patricia Aas’ show at Dotnetrocks:
I have learned that some don’t like code review because it is a critique of the code written. When failing a code review the task is halted for a rewrite and everyone loses.
I love it because to me it is a discussion about code and the system *we* are building *together*.
The code involved is a talking piece.
I prepend my comments with “PRAISE:”, “FIX:”, “SUGGESTION:”, “EXPLAIN:” and “CHECK:”.
FIX is the only one failing a code review.
Depending on the amount of, and severity, EXPLAIN and CHECK might give a red flag too.
The rest are for discussion; to learn and spread knowledge.
PRAISE is important. Only giving negative critique wears a relation. It might also be a part of the reason some don’t like code reviews. It can be hard to recognise good code as, as with many things, it steps out of the way so one does not reflect upon it. Good naming of a method, remember to remove old usings, good test data, …
SUGGESTION is how *I* would like to write the code. Change if you like, don’t if you don’t. I’d be happy to talk about it but there is no need to involve me in a decision.
EXPLAIN on the other hand requires my input. I don’t understand what you are doing – please explain it to me. If it is easy to explain then I should probably have understood; but if you have a hard time explaining it, then there is a good chance the code is too complex. (I have found a bug this way.)
CHECK is more serious. I am not sure you are doing the right thing and would like for you (or anyone else, including me) to double check it.
Typically there will be quite some SUGGESTION followed by a healthy amount of CHECK and EXPLAIN.
Update:
I have used this method for some projects now and only received positive or neutral feedback.
In one project one colleague took every SUGGESTION and implemented them while another ignored anything that wasn’t FIX. Both were skilled and made good choices. We talked a lot about code.
In another project my colleague liked to start with only looking at FIX to get a tally and then read through the rest. S/he is/was junior and we’ve had good discussions about code.
Update:
Someone else has come to the same conclusion: https://conventionalcomments.org/#labels
April 28th, 2017
I have started developing a lib for creating pseudo randomised data. (yes, I know all randomised data on a computer is pseudo randomised, but in my case I want to control the seeding)
The lib, basically, is the ordinary dotnet random class with constructor with some customised constructors to make it easier to add a known seed. It also contains methods for randomising strings with a prefix, a certain length, int, long, decimal, guid, enum and other stuff that is often used.
The seeding is taken from the test method’s name.
This will give the same values for every test run of that method.
February 12th, 2017
Prerequisite
See Compile Aspnet core 1.1 outside Docker container but run within
Create a controller
Create a file TodoController.cs file in the same folder as HomeController.cs file. In a real application we should probably put it in a folder “api” or similar.
Fill it with the text below (which is a refinement of https://docs.microsoft.com/en-us/aspnet/core/tutorials/first-web-api ):
[Update:There is now a
or similar so you don’t have to copypaste the code below. I plan to write about it as soon as I can.]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57
| using System.Collections.Generic;
using System.Linq;
using Microsoft.AspNetCore.Mvc;
namespace WebApplication.Controllers
{
// Setting the Route attribute is important.
// Otherwise we don't get the restish behaviour we're looking for.
// I cannot say exactly why this is so.
[Route("api/[controller]")]
public class TodoController : Controller
{
private static List _data =
new List{
new Item{
Id = 1,
Name = "One"
},
new Item{
Id = 2,
Name = "Two"
},
};
[HttpGet]
public IEnumerable<item> GetAll()
{
return _data;
}
[HttpGet("{id}", Name = "GetTodo")]
public string Get(int id)
{
return _data.Single(d => d.Id == id).Name;
}
[HttpPut("{id}")]
public IActionResult Put(int id, [FromBody] string name)
{
GetItem(id).Name = name;
return new NoContentResult();
}
[HttpPost]
public IActionResult Post(string name)
{
var item = new Item { Id = _data.Count() + 1, Name = name };
_data.Add(item);
return CreatedAtRoute("GetTodo", new { id = item.Id }, item);
}
private Item GetItem(int id)
{
return _data.Single(d => d.Id == id);
}
}
public class Item
{
public int Id { get; set; }
public string Name { get; set; }
}
}
</item> |
Compile:
And start the web server with the application:
1
| dotnet bin/Debug/netcoreapp1.1/MyWs.dll |
Call the controller
Start another terminal.
First check what we have.
1
| curl localhost:5000/api/Todo |
Then check one item.
1
| curl localhost:5000/api/Todo/2 |
Create a new.
1 2
| curl --data "Name=Three" localhost:5000/api/Todo/
curl localhost:5000/api/Todo/3 |
Update an existing according to Stack overflow.
1 2 3 4 5
| curl -H "Content-Type: application/json" \
-X PUT \
--data '"Tva"' \
localhost:5000/api/todo/2
curl localhost:5000/api/Todo/2 |
February 6th, 2017
There is no native image processing lib for dotnet core but someone at Microsoft has been kind enough to list 6 alternatives here, namely CoreCompat.System.Drawing,ImageSharp,Magick.NET, SkiaSharp, FreeImage-dotnet-core and MagicScaler.
January 24th, 2017
We all have this centrally keyboard key that is seldom used. I am thinking of caps lock.
I wanted to use it as an alternative key, not as ctrl or anything; but as something totally different that does not invade existing short cuts.
Rewiring the capslock is harder than it looks at first glance.
I didn’t find a Good solution but at least one that works; for now.
Autohotkey
Autohotkey aka AHK is for writing macros for keyboard, mouse(?) and joystick. The language is awful but don’t let that scare you away.
I have mapped CapsLock to be used with j, k, l and ; to navigate.
Capslock by itself is Esc.
Source code
Regular header stuff in the file:
1 2 3 4
| #NoEnv ; Recommended for performance and compatibility with future AutoHotkey releases.
; #Warn ; Enable warnings to assist with detecting common errors.
SendMode Input ; Recommended for new scripts due to its superior speed and reliability.
SetWorkingDir %A_ScriptDir% ; Ensures a consistent starting directory. |
Make Capslock not work as capslock but also make it work as Esc:
(I believe some magic is involved.)
1 2 3
| SetCapsLockState AlwaysOff
!CapsLock::CapsLock
CapsLock::Send {Esc} |
Map the personal stuff. In my case navigation:
1 2 3 4
| CapsLock & j::Send {Left}
CapsLock & k::Send {Right}
CapsLock & SC027::Send {Down} ; or CapsLock & `;::Send {Down}
CapsLock & l::Send {Up} |
Unfortunately I don’t remember the sources I puzzled together my solution form.
January 14th, 2017
Prerequisite
OSX
Docker
Dotnet core 1.1
A folder named (e.g.) /Users/yourname/Docker/Dandelion
and a folder named (e.g.) /Users/yourname/Docker/Dandelion/MyWeb
Create the web
In MyWeb run
1 2 3 4
| dotnet new -t web
dotnet restore
dotnet build
dotnet publish |
Now we have a web site compiled and ready to run with [dotnet run].
You can run the app and then [curl localhost] to find out if it is runnable; but if you got no error message earlier all should be ok.
Create an image
Go to the Dandelion folder.
Create a file Dockerfile and paste into it:
1 2 3
| FROM microsoft/dotnet
EXPOSE 80
ENV "ASPNETCORE_URLS=http://+:80" |
Then, to create the image run
1
| docker build -t yourname/dandelion . |
Note that the name must be all small caps.
Don’t miss the trailing period.
If you want to check that the image is created just execute [docker images] which should show the new image at the top of the list.
Start the container
Go to the Dandelion folder. (you are probably already standing there)
Execute
1 2 3 4 5
| docker run -p 80:80 \
-ti --rm \
-v /Users/yourname/Documents/Docker/Dandelion/MyWeb/bin/Debug/netcoreapp1.1/publish:/MyWeb \
yourname/dandelion \
/bin/bash -c 'cd /MyWeb; dotnet MyWeb.dll' |
and your web should start.
Verify result
Either open another console and execute
or go to localhost in your web browser.
January 10th, 2017
When trying to
a dotnet core 1.1 project you might receive
1 2
| Publishing TheApp for .NETCoreApp,Version=v1.1
No executable found matching command "npm" |
The remedy is to install NPM.
If you are running OSX you probably got Homebrew with the installation.
So just
Then you might get
No executable found matching command “bower”
which means that Bower is missing. According to https://github.com/dotnet/cli/issues/3293 you should install it through npm like so:
1 2
| npm install -g bower
npm install -g gulp |